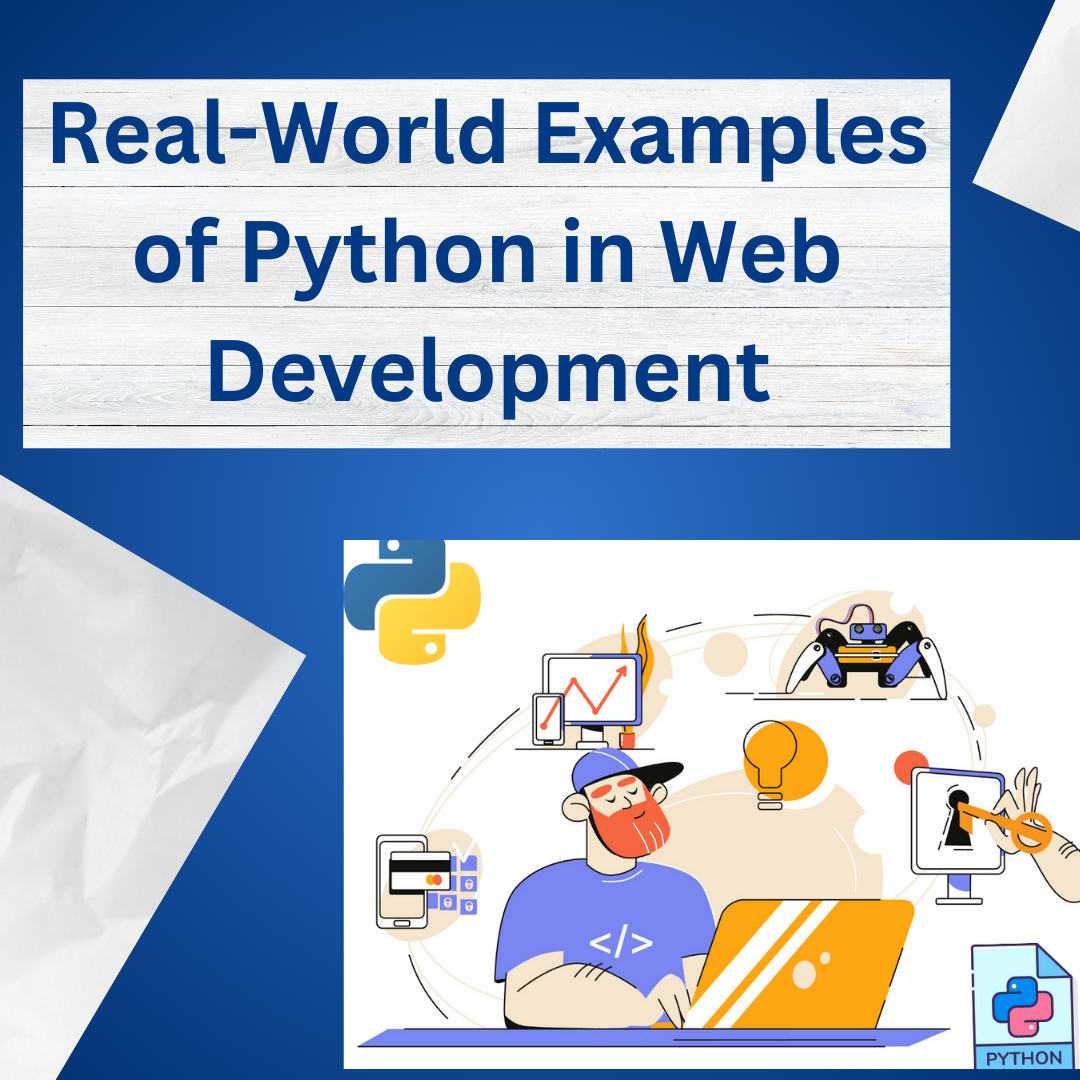
Real-World Examples of Python in Web Development
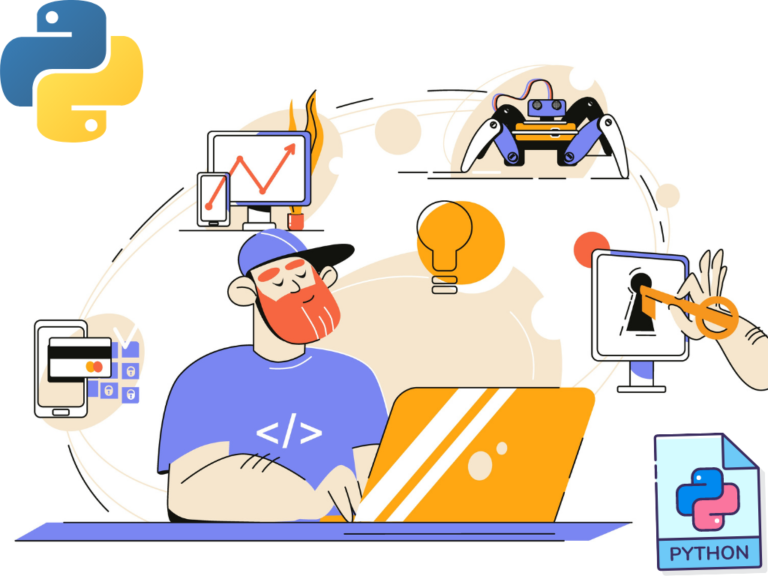
Python has indeed evolved into a versatile and powerful language, garnering widespread acclaim in the field of web development. Its simplicity and readability make it accessible to developers of varying skill levels, fostering collaboration and efficient code maintenance. Python’s strength lies not only in its core features but also in its expansive ecosystem of libraries and frameworks.
Python programming Language stands out for its ability to craft robust and scalable applications. From high-level frameworks like Django, offering a comprehensive suite for rapid development, to micro-frameworks like Flask, providing flexibility for smaller projects, Python caters to a diverse range of needs.
Moreover, emerging technologies such as FastAPI demonstrate how Python seamlessly integrates modern practices, enhancing the efficiency and reliability of web applications. These real-world examples underscore Python’s prowess, showcasing its adaptability and effectiveness in addressing the dynamic challenges of the ever-evolving web development landscape.
Alpha Tech Academy Providing an Exclusive pay after placement program in python full stack developer Course for newly graduated Student to become a full stack developer and get placed in a IT Industry.
1. Django: Building Web Applications Rapidly
Django, a high-level Python web framework, is renowned for its “batteries-included” philosophy, providing everything developers need to build web applications quickly. With a clean and pragmatic design, Django simplifies complex tasks, enabling developers to focus on building features.
Example: Creating a Blog
# models.py
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
# views.py
from django.shortcuts import render
from .models import Post
def blog_home(request):
posts = Post.objects.all()
return render(request, ‘blog/home.html’, {‘posts’: posts})
In this example, Django’s ORM (Object-Relational Mapping) simplifies database interactions, and the built-in template system streamlines HTML rendering, allowing developers to create a fully functional blog with just a few lines of code.
2. Flask: Lightweight and Flexible Web Framework
Flask is a micro-framework that provides the essentials for web development without imposing rigid structures. It’s an excellent choice for smaller projects and startups due to its simplicity and flexibility.
Example: Building an API
from flask import Flask, jsonify
app = Flask(__name__)
@app.route(‘/api/greet/<name>’)
def greet(name):
if __name__ == ‘__main__’:
app.run(debug=True)
if __name__ == ‘__main__’:
app.run(debug=True)
This Flask example illustrates creating a minimal API that greets a user by name. Flask’s lightweight nature allows developers to focus on specific functionalities without unnecessary overhead.
3. FastAPI: Modern API Development
FastAPI has gained popularity for its speed and automatic API documentation generation. It leverages Python type hints for improved code readability and validation, making it an excellent choice for building APIs quickly and efficiently.
Example: CRUD Operations with FastAPI
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
app = FastAPI()
class Item(BaseModel):
name: str
description: str = None
items = []
@app.post(‘/items/’)
def create_item(item: Item):
items.append(item)
return item
@app.get(‘/items/{item_id}’)
def read_item(item_id: int, q: str = None):
if item_id >= len(items):
raise HTTPException(status_code=404, detail=’Item not found’)
return {‘item_id’: item_id, ‘item’: items[item_id], ‘q’: q}
FastAPI’s automatic data validation, as demonstrated in this example, ensures that incoming data adheres to the specified structure, enhancing the reliability of the API
4. Beautiful Soup: Web Scraping Made Easy
Beautiful Soup, a Python library, simplifies the extraction of data from HTML and XML files. It’s a handy tool for web scraping, allowing developers to collect information from websites efficiently.
Example: Extracting Data from a Web Page
import requests
from bs4 import BeautifulSoup
url = ‘https://example.com’
response = requests.get(url)
soup = BeautifulSoup(response.text, ‘html.parser’)
# Extracting titles of articles
titles = [article.text for article in soup.find_all(‘h2′, class_=’article-title’)]
In this example, Beautiful Soup helps extract article titles from a webpage. It showcases Python’s versatility in handling various aspects of web development, including data extraction.
5. Dash: Interactive Web Applications with Data Visualization
Dash, built on top of Flask, enables the creation of interactive web applications for data visualization. It’s particularly useful for developing dashboards and analytics tools.
Example: Creating a Simple Dashboard
import dash
import dash_core_components as dcc
import dash_html_components as html
app = dash.Dash(__name__)
app.layout = html.Div(children=[
html.H1(children=’Sales Dashboard’),
dcc.Graph(
id=’sales-chart’,
figure={
‘data’: [
{‘x’: [1, 2, 3], ‘y’: [10, 5, 8], ‘type’: ‘bar’, ‘name’: ‘Sales’},
],
‘layout’: {
‘title’: ‘Monthly Sales’,
}
}
)
])
if __name__ == ‘__main__’:
app.run_server(debug=True)
Dash simplifies the creation of interactive dashboards. In this example, a sales dashboard with a bar chart is generated with just a few lines of code.
Conclusion
Python’s presence in web development is pervasive, thanks to its readability, extensive libraries, and frameworks catering to diverse needs. Whether you’re building full-stack web applications, APIs, scraping data, or creating interactive dashboards, Python provides a versatile and user-friendly environment for developers. These real-world examples showcase Python’s effectiveness in addressing the challenges of modern web development with simplicity and clarity.
Why Join Python Full Stack Developer Course in Alpha Tech Academy?
Joining the Python Full Stack Developer course at Alpha Tech Academy is like opening a door to a super useful learning adventure. In this course, you get to learn everything about Python and how to use it to build awesome websites. The teachers are really experienced and make sure you understand things in a way that’s easy.
At Alpha Tech Academy, they don’t just teach or talk about coding, they let you do it! You’ll work on cool projects that show you exactly how things work in the real world. Plus, they help you find great job opportunities after you finish the course.
So, if you want to be really good at using Python to build all sorts of things on the internet, joining the Python Full Stack Developer course at Alpha Tech Academy is a smart move. It’s like learning the secrets of web development in a way that’s fun and super easy to understand! And this course is 100% Pay After Placement. Don’t Miss It!
Check Python Full Stack Developer Salary Detail in India – https://www.ambitionbox.com/profile/python-fullstack-developer-salary