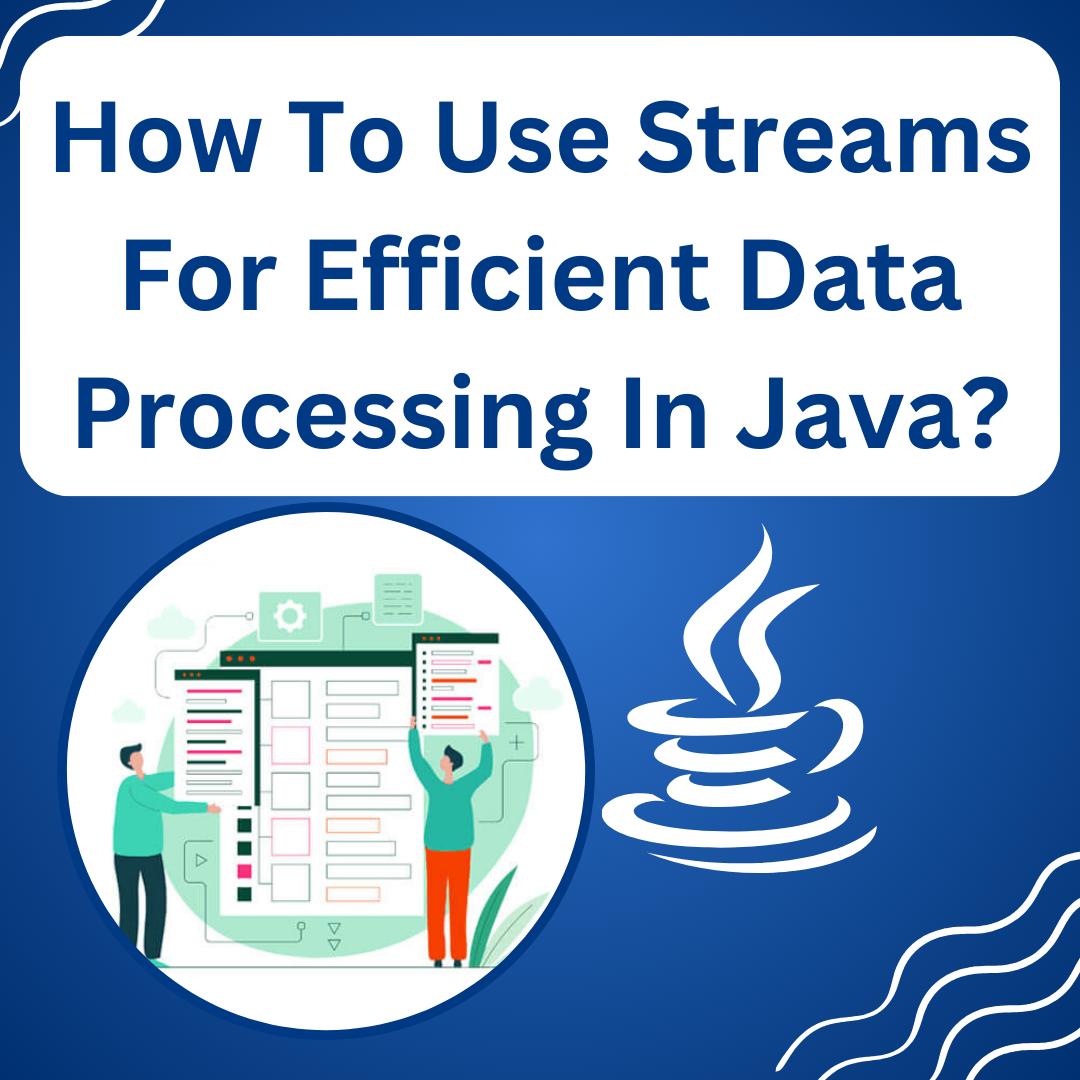
How To Use Streams For Efficient Data Processing In Java?
Mastering efficient data processing is a key skill for developers. One powerful tool at your disposal is the Java Stream API. Streams provide a concise and expressive way to perform operations on data, making your code more readable and maintainable. In this blog, we’ll explore how to harness the power of streams for efficient data processing in Java.
To become a java fullstack developer join a pay after placement course and secure your job in the IT Industry.
Understanding Java Streams
Before diving into practical examples, let’s understand what Java Streams are. In simple terms, a stream is a sequence of elements that can be processed in parallel or sequentially. It does not store data but provides a pipeline for data manipulation.
Creating Streams
You can create streams from various sources like collections, arrays, or even generate them using Stream API methods. Here’s a quick example:
List<String> fruits = Arrays.asList(“Apple”, “Banana”, “Orange”, “Grapes”);
// Creating a stream from a List
Stream<String> fruitStream = fruits.stream();
Stream Operations
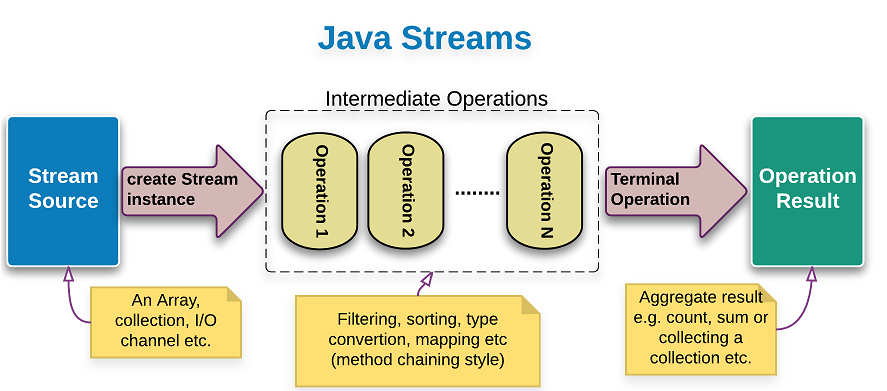
Once you have a stream, you can perform various operations on it. There are two types of operations: intermediate and terminal.
- Intermediate Operations: These operations transform a stream into another stream. Examples include filter, map, and sorted.
// Filtering fruits starting with ‘A’
Stream<String> filteredFruits = fruitStream.filter(fruit -> fruit.startsWith(“A”));
Terminal Operations: These operations produce a result or side-effect. Examples include forEach, collect, and reduce.
// Printing filtered fruits
filteredFruits.forEach(System.out::println);
Simplifying Data Filtering with Streams
Now, let’s consider a practical scenario where you have a list of numbers, and you want to filter out the even ones. Without streams, you might use a loop like this:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
// Filtering even numbers without streams
List<Integer> evenNumbers = new ArrayList<>();
for (Integer number : numbers) {
if (number % 2 == 0) {
evenNumbers.add(number);
}
}
With streams, you can achieve the same result more succinctly:
// Filtering even numbers with streams
List<Integer> evenNumbers = numbers.stream()
.filter(number -> number % 2 == 0)
.collect(Collectors.toList());
By using streams, you eliminate the need for explicit iteration and temporary collections, making your code cleaner and easier to understand.
Transforming Data with Stream Mapping
Another common use case is transforming elements in a collection. Consider a scenario where you have a list of words, and you want to convert them all to uppercase:
List<String> words = Arrays.asList(“hello”, “world”, “java”, “stream”);
// Converting words to uppercase without streams
List<String> uppercaseWords = new ArrayList<>();
for (String word : words) {
uppercaseWords.add(word.toUpperCase());
}
Using streams and the map operation, you can achieve the same result more elegantly:
// Converting words to uppercase with streams
List<String> uppercaseWords = words.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
Combining Operations for Complex Tasks
One of the strengths of streams is the ability to chain multiple operations together, creating a concise and expressive flow. Let’s say you have a list of transactions, and you want to find the total value of transactions made by a specific user:
List<Transaction> transactions = Arrays.asList(
new Transaction(“user1”, 100),
new Transaction(“user2”, 150),
new Transaction(“user1”, 200),
new Transaction(“user3”, 50)
);
// Finding the total value of transactions for ‘user1’ without streams
int totalValue = 0;
for (Transaction transaction : transactions) {
if (“user1”.equals(transaction.getUser())) {
totalValue += transaction.getValue();
}
}
Using streams, you can achieve the same result more efficiently:
// Finding the total value of transactions for ‘user1’ with streams
int totalValue = transactions.stream()
.filter(transaction -> “user1”.equals(transaction.getUser()))
.mapToInt(Transaction::getValue)
.sum();
By combining filter, map, and sum operations, you create a concise and readable solution.
Conclusion
Java Streams provide a powerful and expressive way to perform efficient data processing. By leveraging streams, you can write code that is more concise, readable, and maintainable. Whether you’re filtering, mapping, or combining operations, streams offer a versatile toolkit for handling data in a streamlined manner.
As you explore deeper into Java development, mastering streams will enhance your ability to write clean and efficient code. Practice incorporating streams into your projects, and soon you’ll find yourself appreciating the elegance they bring to data processing in Java.
Why Join Java Full Stack Developer Course at Alpha Tech Academy ?
Embarking on a Java fullstack developer course at Alpha Tech Academy offers a gateway to a rewarding career in the IT industry. Here are compelling reasons why enrolling in this course can pave the way for success in your professional journey.
Comprehensive Curriculum: Alpha Tech Academy’s Java full-stack course is meticulously designed, covering both front-end and back-end technologies. From mastering Java programming to diving into advanced web development frameworks, you gain a holistic understanding of the full-stack ecosystem.
Experienced Instructors: Learn from seasoned industry professionals with real-world experience. The instructors at Alpha Tech Academy bring a wealth of knowledge, ensuring that you receive practical insights into the latest industry trends and best practices.
Hands-On Projects: The course emphasizes practical learning through hands-on projects. By working on real-world applications, you not only solidify theoretical concepts but also build a robust portfolio that showcases your skills to potential employers.
Industry-Relevant Tools and Technologies: Stay ahead in the rapidly evolving tech landscape by gaining proficiency in cutting-edge tools and technologies. Alpha Tech Academy keeps its curriculum updated, ensuring that you are well-versed in the tools currently in demand within the IT industry.
Placement Assistance: Upon successful completion of the course, you benefit from Alpha Tech Academy’s dedicated placement assistance. The academy maintains strong connections with industry leaders, facilitating opportunities for interviews and job placements, giving you a head start in your IT career.
Networking Opportunities: Joining the Java full-stack course at Alpha Tech Academy opens doors to a vibrant community of like-minded learners. Networking events, workshops, and alumni interactions create valuable connections that may prove instrumental in your career growth.
Enrolling in the Java full-stack course at Alpha Tech Academy not only equips you with the skills demanded by the IT industry but also provides a supportive environment for your professional development.
With a comprehensive curriculum, experienced instructors, hands-on projects, and strong placement support, this course sets the stage for a successful and fulfilling career in the dynamic field of IT.