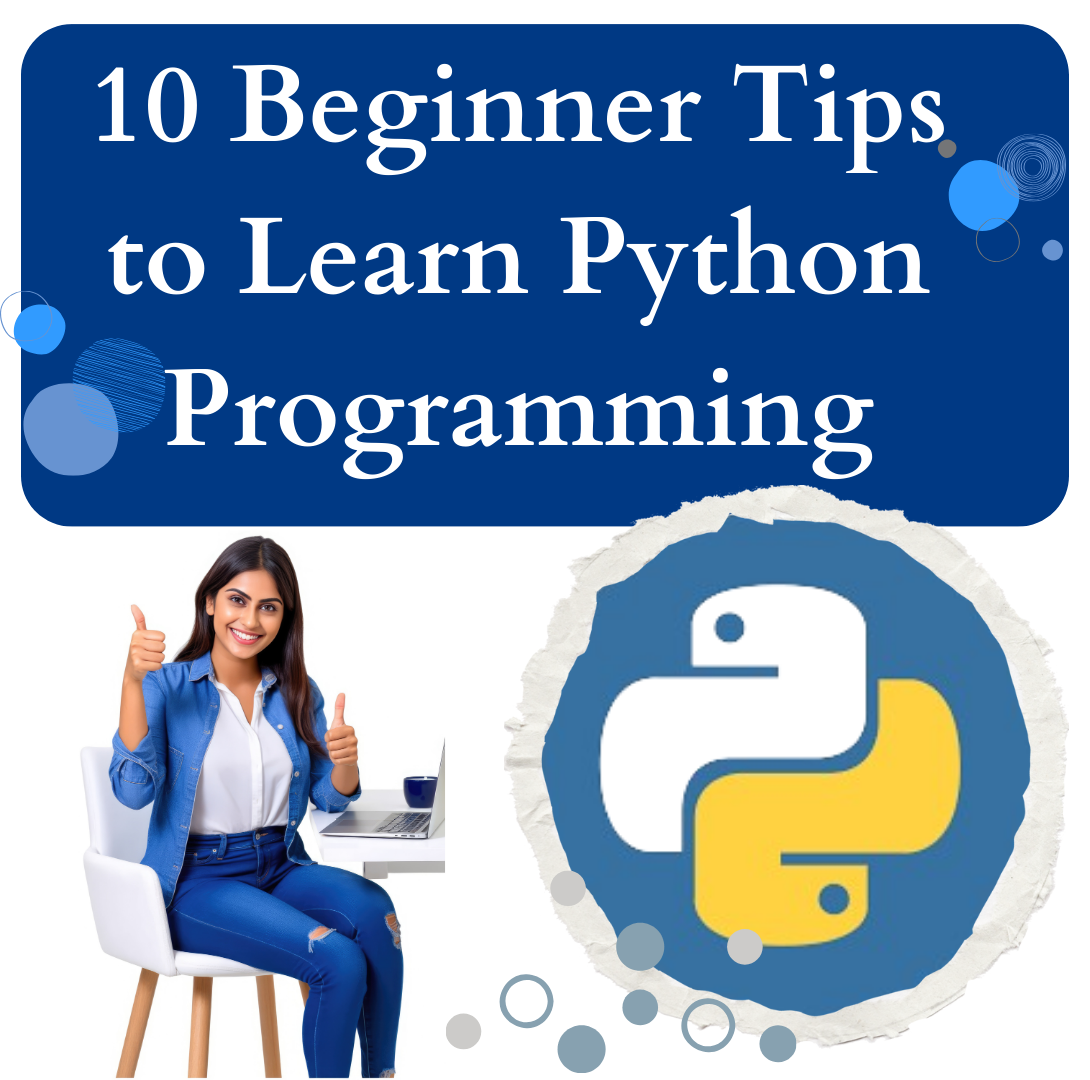
10 Beginner Tips To Learn Python Programming
We’re excited that you’ve chosen to begin your Python learning journey! A question we frequently encounter is, “What’s the most effective way to learn Python programming?”.
We firmly believe that the initial step in mastering any programming language is understanding how to learn effectively. This skill is arguably the most crucial aspect of computer programming.
But why is learning how to learn so vital? It’s straightforward: languages evolve, libraries emerge, and tools advance. Being adept at learning will be essential for staying abreast of these changes and excelling as a programmer.
In this blog, we’ll present various learning strategies to kickstart your path to becoming a proficient Python programmer!
Make It Stick
Here are some tips to help beginner programmers solidify the new concepts they are learning:
Tip #1: Code Everyday
Maintaining consistency is crucial when embarking on learning a new language. We’d like to suggest that you dedicate yourself to coding daily. Surprisingly, muscle memory significantly influences programming. Engaging in coding every day will effectively cultivate this muscle memory. While it might feel overwhelming initially, consider beginning with a modest commitment, such as 25 minutes daily, and gradually increasing your practice time.
If you would like guidance on setup and introductory exercises, you can refer to the First Steps With Python Guide.
Tip #2: Write It Out
Whether you’re just starting to learn Python programming basic data structures like strings, lists, and dictionaries, or you’re troubleshooting an application, the interactive Python shell is an invaluable learning tool. We also frequently use it on this site!
To access the interactive Python shell (sometimes called a “Python REPL”), start by ensuring Python is installed on your computer. If not, you can follow our step-by-step tutorial to install it. Once Python is installed, simply open your terminal and type either python or python3, depending on your installation. For more detailed instructions, you can refer to our specific directions.
Tip #3: Go Interactive!
Whether you’re new to learning about basic Python data structures like strings, lists, and dictionaries, or you’re fixing issues in an application, the interactive Python shell is a fantastic tool for learning. It’s something we often use here too!
To use the interactive Python shell (also known as a “Python REPL”), start by confirming that Python is installed on your computer. If you’re unsure, we have a step-by-step tutorial to guide you through the installation process. Once Python is installed, simply open your terminal and type either python or python3, depending on your setup, to activate the interactive Python shell.
Now that you’re familiar with launching the shell, here are some examples of how you can utilize it for learning:
Learn what operations can be performed on an element by using dir():
>>> my_string = ‘I am a string’
>>> dir(my_string)
[‘__add__’, …, ‘upper’, ‘zfill’] # Truncated for readability
The results obtained from using dir() consist of all the methods (or actions) that you can apply to the element. For instance:
>>> my_string.upper()>> ‘I AM A STRING’
Did you notice when we used the upper() method? Do you know what it does? It converts all the letters in the string to uppercase! To explore more about these built-in methods, check out the section on “Manipulating strings” in this tutorial.
Learn the type of an element:
>> type(my_string)
>> str
Use the built-in help system to get full documentation:
>> help(str)
Import libraries and play with them:
>>> from datetime import datetime
>>> dir(datetime)
[‘__add__’, …, ‘weekday’, ‘year’] # Truncated for readability
>>> datetime.now()
datetime.datetime(2018, 3, 14, 23, 44, 50, 851904)
Run shell commands:
>>> import os
>>> os.system(‘ls’)
python_hw1.py python_hw2.py README.txt
Tip #4: Take Breaks
When you’re learning, it’s crucial to take breaks to let new concepts sink in. The Pomodoro Technique is great for this: you work for 25 minutes, then take a short break before starting again. This method helps you absorb information effectively.
Breaks are especially important when debugging. If you’re stuck on a problem, stepping away can help. Take a walk or talk to a friend to clear your mind.
In programming, tiny mistakes like missing a quotation mark can cause big problems. Taking a break and coming back with fresh eyes can help you catch these errors.
Tip #5: Become a Bug Bounty Hunter
When you’re coding complex programs, encountering bugs is normal. It happens to everyone! Instead of getting frustrated, think of yourself as a bug bounty hunter and tackle these challenges with pride.
When debugging, it’s helpful to follow a step-by-step approach. Go through your code in the order it’s executed, checking each part to ensure it works properly.
If you have an idea of where the problem might be, try adding this line of code to your script: import pdb; pdb.set_trace(). Then run your script. This activates the Python debugger, which lets you interactively inspect your code. You can also run the debugger from the command line using python -m pdb <my_file.py>
Tip #6: Surround Yourself With Others Who Are Learning
Even though coding can feel like something you do alone, it’s actually better when you work with others. When you’re learning Python, it’s really important to be around other people who are also learning. This way, you can share what you’re learning and pick up tips and tricks from each other.
If you don’t know anyone who is into coding, don’t worry! There are lots of ways to meet people who love Python too! You can join local events or Meetups, or check out online communities like PythonistaCafe. It’s a place where people who love Python help each other learn and grow.
Tip #7: Teach
They say that one of the best ways to learn something is to teach it to others. This applies to learning Python too. There are different ways you can do this: you can solve problems on a whiteboard with fellow Python enthusiasts, write blog posts to explain what you’ve learned, make videos where you teach others, or even just talk through concepts by yourself at your computer. All of these methods help reinforce your understanding and show you where you might need to learn more.
Tip #8: Pair Program
Pair programming is when two developers work together at one computer to finish a task. They take turns being the “driver,” who writes the code, and the “navigator,” who helps guide the problem-solving and reviews the code. It’s important to switch roles often to get the benefits of both perspectives.
Pair programming has lots of advantages: it lets you not only have someone check your code but also see how they approach problem-solving. Being exposed to different ideas and ways of thinking will help you become better at solving problems when you’re coding on your own.
Tip #9: Ask “GOOD” Questions
When you’re asking for help in programming, it’s important to ask good questions. This means providing context about the problem you’re facing, outlining what you’ve already tried, offering your best guess about the problem, and demonstrating what’s happening with your code, including any error messages and the steps you took. Good questions can save time and prevent conflicts. As a beginner, practicing asking good questions helps you communicate effectively and encourages others to continue helping you.
Make Something
Many Python developers agree that the best way to learn Python is by actually making things. While doing exercises is helpful, the most effective learning happens when you start building projects.
Tip #10: Build Something, Anything
For beginners, doing small exercises can really boost your confidence with Python and help you build up your coding skills. Once you’ve got a good handle on basic concepts like strings, lists, dictionaries, sets, object-oriented programming, and writing classes, it’s time to start creating your own projects!
The specific projects you choose aren’t as important as the process of building them. The journey of creating something is where you’ll learn the most. While reading articles and taking courses can be helpful, the majority of your learning will come from actually using Python to build things and solving problems along the way.
There are plenty of lists out there with ideas for beginner Python projects. Here are a few suggestions to get you started:
- A number guessing game
- A simple calculator app
- A dice roll simulator
- A Bitcoin price notification service.
If you’re struggling to come up with project ideas, check out this video. It offers a strategy you can use to generate thousands of project ideas whenever you’re feeling stuck.