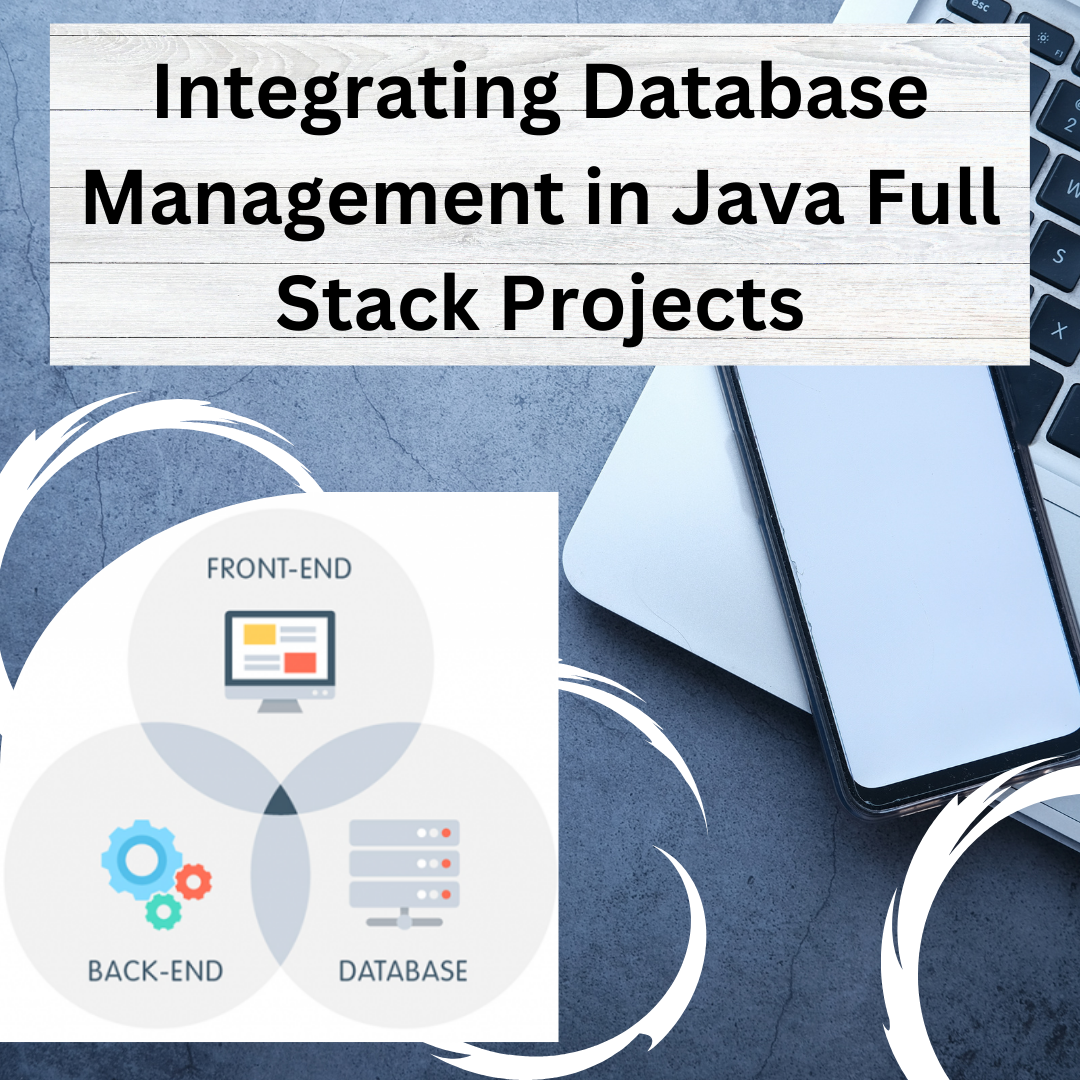
Integrating Database Management in Java Full Stack Projects
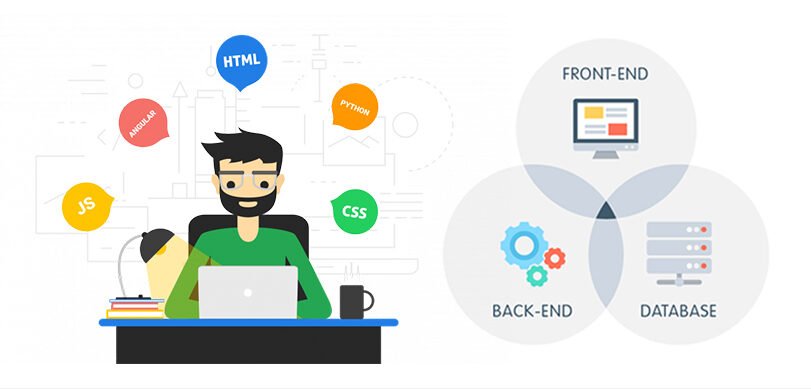
In Java Full Stack development, understanding how to seamlessly integrate database management is similar to discovering the secret treasure that is unforgettable. Whether you’re crafting or developing a robust application, every process (or in our case, every piece of data) needs to be perfectly aligned and integrated.
Venturing into the tech world, grasping the complexities of database integration is important. This guide will walk you through the best practices for combining SQL and NoSQL databases with Java applications, ensuring your projects are not just functional but also scalable and efficient.
Understanding Database Integration
In Java Full Stack development, understanding how to seamlessly integrate database management is similar to discovering the secret treasure that is unforgettable. Whether you’re crafting or developing a robust application, every process (or in our case, every piece of data) needs to be perfectly aligned and integrated.
Venturing into the tech world, grasping the complexities of database integration is important. This guide will walk you through the best practices for combining SQL and NoSQL databases with Java applications, ensuring your projects are not just functional but also scalable and efficient.
1. Choosing the Right Database
- SQL Databases: SQL (Structured Query Language) databases, like MySQL, PostgreSQL, and Oracle, are akin to well-organized libraries. They’re perfect for applications requiring complex transactions and where data integrity is non-negotiable.
- NoSQL Databases: NoSQL databases, such as MongoDB, Cassandra, and CouchDB, offer flexibility and scalability. They’re the go-to for applications dealing with large volumes of unstructured data or requiring rapid scaling.
2. JDBC: The Bridge Builder
Java Database Connectivity (JDBC) is your first tool in building the bridge. It’s a Java API that connects Java applications to a wide range of databases. Using JDBC, you can execute SQL queries, update data, and retrieve results—all within your Java application. It’s like having a universal key to access any library in the city.
- Cross-Platform Capability: One of the standout features of JDBC is its cross-platform capability. This means you can run your Java application on any operating system, be it Windows, Mac, Linux, or any other, and still maintain a stable connection to your database. It ensures that your application is versatile and adaptable, ready to meet the demands of a diverse user base.
- Driver Types for Flexibility: JDBC offers various types of drivers that facilitate connection to different databases. Whether you’re connecting to a local database or a database server over the network, there’s a JDBC driver tailored for your needs. This flexibility allows developers to choose the most efficient driver based on their project’s requirements, optimizing performance and resource utilization.
- Batch Processing for Efficiency: JDBC supports batch processing, allowing you to group multiple SQL statements and execute them as a batch. This reduces the number of round trips between the application and the database, significantly improving the performance of your application. Batch processing is particularly beneficial when you need to insert or update large volumes of data simultaneously, making your database interactions more efficient and faster.
Incorporating these additional points, JDBC not only acts as a bridge but also enhances the efficiency, flexibility, and adaptability of Java applications interacting with databases. It’s a powerful tool in the collection of Java Full Stack development, enabling them to build robust and high-performance applications.
3. Spring Data JPA: Simplifying Database Operations
For those who prefer a higher level of abstraction, Spring Data JPA (Java Persistence API) comes to the rescue. It simplifies data access operations, reducing the boilerplate code required for JDBC. Think of it as hiring a librarian who knows exactly where every book is placed and can fetch them for you without you having to navigate the labyrinthine aisles.
4. Integrating NoSQL Databases
When it comes to NoSQL databases, Spring Data provides specific modules like Spring Data MongoDB, Spring Data Cassandra, etc. These modules make it a breeze to integrate NoSQL databases into your Java application, ensuring you can focus more on your application’s logic rather than the nitty-gritty of database operations.
- Polyglot Persistence: Spring Data supports using multiple database types within the same application. This flexibility enables leveraging specific strengths of different databases, enhancing application scalability and performance.
- Repository Abstraction: Spring Data offers a repository abstraction layer that minimises CRUD operation boilerplate. This speeds up development by automating underlying implementations, letting developers concentrate on business logic.
These points underscore how Spring Data enhances NoSQL database integration, offering both flexibility in database choice and efficiency in development.
5. Best Practices for Database Integration
- Database Normalization: Ensure your SQL database is normalized to eliminate redundancy and improve data integrity. It’s like organizing your bookshelf so that every book is easy to find and there’s no unnecessary duplication.
- Indexing: Implement indexing in both SQL and NoSQL databases to speed up query processing. Think of it as creating a detailed index at the beginning of a textbook, helping you quickly locate the information you need.
- Connection Pooling: Use connection pooling to manage database connections efficiently. It’s similar to having a pool of ready-to-use bicycles at your college, ensuring you always have one available when you need it.
6. Testing Your Database Integration
For those who prefer a higher level of abstraction, Spring Data JPA (Java Persistence API) comes to the rescue. It simplifies data access operations, reducing the boilerplate code required for JDBC. Think of it as hiring a librarian who knows exactly where every book is placed and can fetch them for you without you having to navigate the labyrinthine aisles.
7. Handling Data Migration
Data migration is an essential aspect of database management. Tools like Flyway and Liquibase can automate database version control and migrations, making it easy to update your database schema without breaking a sweat.
Conclusion
Integrating database management in Java Full Stack projects might seem daunting at first, but with the right tools and practices, it becomes an exciting adventure. Remember, every great application starts with a solid foundation, and understanding how to effectively integrate databases is a crucial part of that foundation. So, to all the aspiring Java Full Stack developers in India, embrace these practices, experiment, and don’t be afraid to make mistakes. After all, every coding challenge you overcome adds a new flavor to your development journey, making you not just a coder, but a craftsman of the digital age.